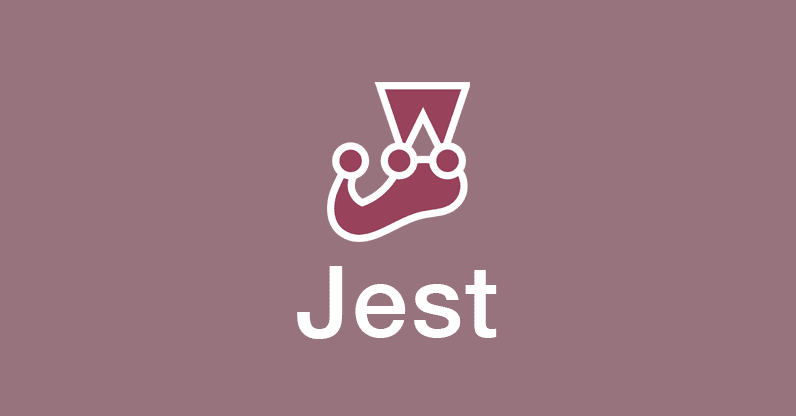
Jest is a JavaScript testing framework that makes it easy to test and validate the functionality of JavaScript code. It is a popular choice among developers and QA engineers for its simplicity, speed, and robust feature set. Jest provides a comprehensive set of testing tools, including support for unit, integration, and end-to-end testing. Jest also integrates with other popular JavaScript libraries and frameworks, making it a versatile and flexible solution for any JavaScript testing needs. With its straightforward syntax and intuitive API, Jest makes it easy for developers and QA engineers to get started with testing quickly and efficiently.
What is Jest?
Jest is a JavaScript testing framework created by Facebook. It is used to create automated tests for JavaScript applications. Jest is a popular choice for testing JavaScript code, as it is fast and easy to use. It is also open source, meaning it is free to use and modify. Jest is used by many companies, including Airbnb, Twitter, and Uber.
Jest is designed to make testing simpler and more efficient. It is built on top of Jasmine, another popular JavaScript testing framework. Jest makes it easier to create tests, as it provides a built-in test runner and assertion library. It also offers mocking capabilities, which makes it easier to unit-test components.
Jest allows developers to write tests in a concise and readable syntax. It provides a set of built-in assertions that make it easy to verify that the code is working as expected. Jest also makes writing tests for asynchronous code easy, as it provides a way to wait for promises to be resolved before continuing with the test.
Advantages of Jest
Jest provides many advantages compared to other testing frameworks. Here are five benefits of Jest that make it stand out from the competition.
1. Easy to Setup and Use
One of the biggest advantages of Jest is that it’s incredibly easy to set up and use. All you have to do is install it, configure it, and start writing tests. This makes it ideal for developers who are new to testing and those who don’t have much time to set up and learn a new testing framework.
2. Automated Testing
Jest also offers automated testing, a great advantage for developers who need to quickly and accurately test their code. With automation testing, developers can quickly identify and fix any bugs or errors before their code is deployed. This saves time and ensures that the code is as bug-free as possible.
3. Cross-Platform Support
Jest also offers cross-platform support, which can be used on multiple platforms. This makes it a great choice for developers who want to ensure that their code is compatible with various platforms.
4. Easy to Maintain
Jest also offers easy maintenance, a huge advantage for developers dealing with a large codebase. With Jest, developers can easily update and maintain their tests, making it easier to keep their code up to date.
5. Fast Performance
Finally, Jest also offers fast performance. This means that developers can quickly and efficiently test their code, which is especially important when dealing with large and complex codebases.
Features Offered by Jest
Jest provides many features that make it a powerful and easy-to-use testing framework. Its built-in test runner, assertion library, and mocking library are the most notable features.
- The built-in test runner allows developers to write and run tests quickly and easily. It also provides features such as test coverage reports, which make it easy to identify areas of code that are not being tested.
- The assertion library provides a set of built-in assertions that make it easy to verify that the code is working as expected. It also provides a way to snapshot tests, allowing developers to easily compare test results to a known good version easily.
- The mocking library allows developers to mock out components for unit testing. It also provides a way to wait for promises to be resolved before continuing the test. This makes it easier to write tests for asynchronous code.
Tests You Can Run on Jest
1. Unit Tests
These are the most basic type of tests used to test the functionality of individual units of code. Unit tests verify that a piece of code works as expected and that any changes made won’t break existing functionality. Unit tests are usually written in isolation and are easy to maintain and refactor. Jest makes it easy to write unit tests with its built-in assertion library and mocking capabilities.
2. Integration Tests
These tests are used to test how different units of code interact with each other. These tests are more complex than unit tests and often require more setups as they involve multiple components. Jest makes it easy to write integration tests with built-in mocking capabilities and test runners.
3. End-to-End (E2E) Tests
These tests are used to test how an application behaves in its entirety. These tests simulate how a user would interact with the application and verify that the application behaves as expected. Jest makes it easy to write end-to-end tests with its built-in test runners and assertion library.
4. Snapshot Tests
These tests are used to test how a component renders. They take a “snapshot” of a component’s output and then compare it to a stored reference snapshot. If the output changes, the test will fail. Jest makes it easy to write snapshot tests with its built-in snapshot testing feature.
5. Performance Tests
These are used to test how an application performs under different conditions. These tests simulate different workloads and measure how the application responds regarding speed and stability. Jest makes it easy to write performance tests with its built-in performance testing feature.
These are the most common types of tests you can run with Jest. Each type of test has its own strengths and weaknesses, and it’s important to understand the differences between them in order to choose the right type of test for your project.
Jest Vocabulary
When working with Jest, it is important to understand the various terms and concepts associated with it. Here are some of the most common terms used when working with Jest:
- Test: A test is a code block that verifies the behavior of a particular piece of code. Tests are written in JavaScript and can be executed by the Jest test runner.
- Assertions: Assertions are statements that verify that the code is behaving as expected. Jest provides a set of built-in assertions that make it easy to verify that the code is working as expected.
- Mocking: It is a process of substituting a real component with a fake one. Jest provides a mocking library that makes it easy to mock out components for unit testing.
- Snapshot Testing: It is a feature of Jest that allows developers to save a “snapshot” of a test result and compare it to a known good version. This is useful for verifying that code is working as expected.
How To Get Started with Jest
Now that you understand the basics of Jest, you can get started writing and running your own tests. Here are the steps you need to take to get started with Jest:
Step 1: Install Jest
The first step in running tests in Jest is to install the framework. Jest is distributed as an npm package, so you can install it with the following command:
npm install –save-dev jest
Step 2: Configure Jest
The next step is to configure Jest. Jest’s configuration is contained in a file called jest.config.js, which is located at the root of your project. In this file, you can specify the test environment, the setup files for each test, and the test files that should be run. You can also configure Jest to run tests in parallel, significantly reducing the time it takes to run tests.
Step 3: Write Tests
Once Jest is configured, it’s time to write your tests. Jest provides a few different ways to write tests. The most common way is to use the describe() and it() functions. The describe() function is used to group related tests together, while it() function is used to define individual tests. For example, the following code defines a test that checks if a function returns the expected value:
describe(‘myFunction()’, () => {
it(‘should return the expected value’, () => {
expect(myFunction()).toEqual(expectedValue);
});
});
Step 4: Run Tests
Once you’ve written your tests, you’re ready to run them. Jest provides a few different ways to run tests. You can run all tests in your project with the following command:
jest
Or, if you want to run an individual test, you can use the following command:
jest myTestFile.test.js
Step 5: Analyze Test Results
Once Jest has run your tests, it will generate a report that contains information about the test results. This report includes the number of tests that were run, the number of tests that passed, and the number of tests that failed. It also includes information about any errors that were encountered during the test run. This information can be used to identify any issues that need to be addressed before the application is ready for deployment.
Conclusion
In conclusion, Jest is a powerful and efficient JavaScript testing framework that offers a range of advantages and features to developers and QA engineers. With its simple and intuitive API, Jest provides a high level of flexibility and ease of use, making it a popular choice for novice and experienced testers. Its robust assertion library, mocking capabilities, and snapshot testing features make Jest an ideal choice for testing modern JavaScript applications. Additionally, Jest’s ability to run tests in parallel and its built-in code coverage analysis tools help to improve test performance and reduce the time required to complete tests. Whether you are new to testing or an experienced professional, Jest offers a comprehensive solution for all your JavaScript testing needs.
In addition to its features and benefits, Jest testing can be even more valuable when combined with the capabilities of LambdaTest. LambdaTest provides a cloud-based platform that enables developers and QA engineers to perform cross-browser testing on a wide range of browsers and operating systems. This means that Jest tests can be run on various environments, giving teams greater confidence in the compatibility and performance of their applications. Furthermore, LambdaTest’s real-time testing and debugging tools provide a more efficient and streamlined testing process, helping teams to identify and fix issues faster. With the combined power of Jest and LambdaTest, developers and QA engineers have a powerful and flexible solution for all their testing needs.