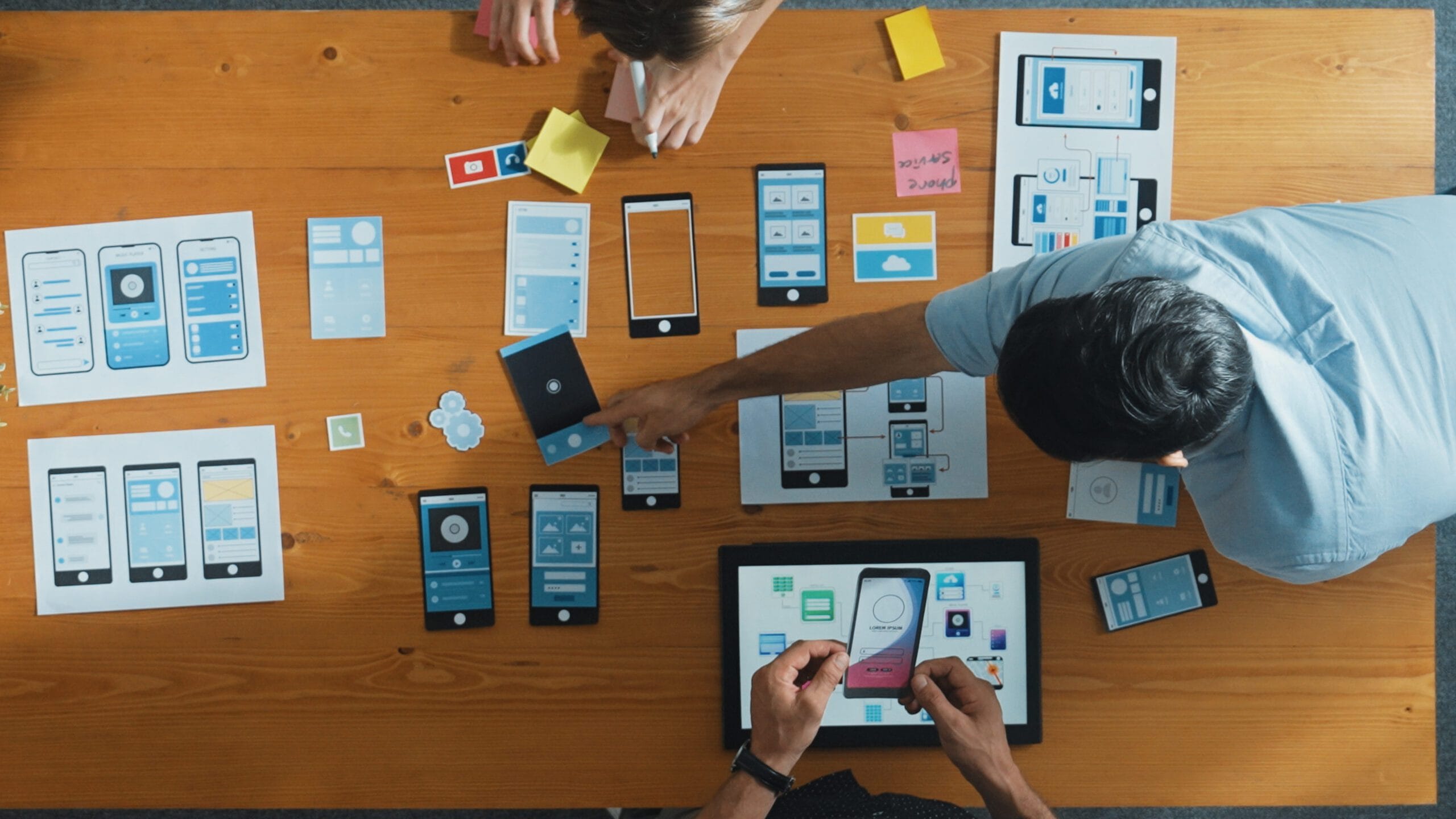
In today’s competitive mobile landscape, ensuring that your Android app delivers a fast and responsive user experience is paramount. Users expect seamless interactions, quick load times, and smooth transitions, all of which contribute to the overall success and reputation of your application. Whether you’re a seasoned developer or just starting with Android app development, optimizing performance should be a top priority. This blog explores actionable tips to enhance the performance of your Android apps, ensuring they run efficiently and provide an exceptional user experience.
1. Efficient Layout Design
One of the fundamental aspects of a performant Android app is the efficiency of its layout design. Complex and deeply nested layouts can significantly slow down your app’s rendering time. To optimize layout performance:
- Use ConstraintLayout: This layout allows you to create complex interfaces without the need for multiple nested layouts, reducing the view hierarchy’s depth.
- Minimize View Hierarchies: Strive for a flat view hierarchy by avoiding unnecessary nesting of views. Tools like the Layout Inspector can help identify and simplify complex hierarchies.
- Leverage ViewStub: For views that are not immediately visible, use ViewStub to inflate them only when needed, saving initial loading time.
2. Optimize Image Resources
Images often consume a significant portion of an app’s resources. Optimizing how images are handled can lead to substantial performance gains:
- Use Appropriate Image Formats: Choose the right image format (e.g., WebP for better compression without quality loss) to reduce file sizes.
- Implement Lazy Loading: Load images only when they are about to appear on the screen using libraries like Glide or Picasso. This approach minimizes memory usage and speeds up initial loading times.
- Resize Images Appropriately: Scale images to match the display size required by your app, preventing unnecessary memory consumption.
3. Efficient Memory Management
Proper memory management is crucial to prevent leaks and ensure smooth app performance:
- Avoid Memory Leaks: Use tools like LeakCanary to detect and fix memory leaks. Ensure that activities, fragments, and other components release resources appropriately.
- Use Weak References: When holding references to large objects or contexts, use weak references to allow garbage collection when needed.
- Optimize Bitmap Usage: Reuse bitmap memory where possible and consider using bitmap pooling to manage memory more efficiently.
4. Asynchronous Programming
Performing tasks on the main thread can lead to unresponsive UI and poor user experiences. Implement asynchronous programming to handle long-running operations:
- Use Kotlin Coroutines: Coroutines simplify asynchronous programming, allowing you to write non-blocking code that is easy to read and maintain.
- Leverage WorkManager: For background tasks that need guaranteed execution, WorkManager provides a reliable API that works seamlessly with the Android lifecycle.
- Implement AsyncTask (With Caution): While deprecated in newer Android versions, AsyncTask can still be useful for simple asynchronous operations when used carefully.
5. Optimize Network Operations
Network requests can be a major source of delays if not handled efficiently:
- Use Caching: Implement caching strategies to reduce redundant network calls. Libraries like Retrofit combined with OkHttp can help manage caching effectively.
- Minimize Data Transfer: Compress data and use efficient data formats like JSON or Protocol Buffers to reduce the amount of data transferred over the network.
- Handle Network Responses Efficiently: Parse network responses asynchronously and handle errors gracefully to prevent blocking the main thread.
6. Implement Code Profiling and Monitoring
Regular profiling and monitoring are essential to identify and address performance bottlenecks:
- Use Android Profiler: Integrated into Android Studio, the Android Profiler provides real-time data on CPU, memory, network, and energy usage, helping you pinpoint performance issues.
- Leverage Third-Party Tools: Tools like Firebase Performance Monitoring offer insights into app performance in real-world scenarios, allowing you to track and improve user experiences continuously.
- Conduct Regular Testing: Perform performance testing on various devices and configurations to ensure consistent performance across the board.
7. Optimize Database Operations
Efficient database management can significantly enhance app performance, especially for data-intensive applications:
- Use Room Persistence Library: Room provides an abstraction layer over SQLite, making database operations more efficient and less error-prone.
- Perform Database Operations Off the Main Thread: Ensure that all database interactions occur on background threads to prevent UI freezes.
- Index Database Tables: Proper indexing can speed up query execution times, especially for large datasets.
8. Reduce App Size
A smaller app size leads to faster downloads and installations, as well as better performance:
- Enable ProGuard/R8: These tools obfuscate and optimize your code, removing unused classes and methods to reduce the app size.
- Use Dynamic Feature Modules: Modularize your app to include features only when needed, allowing users to download parts of the app on demand.
- Optimize Resource Usage: Remove unused resources and compress assets to minimize the overall size of your app.
9. Leverage Hardware Acceleration
Utilizing hardware acceleration can offload rendering tasks to the device’s GPU, improving performance:
- Enable Hardware Acceleration: By default, hardware acceleration is enabled for applications, but ensure that it’s not disabled in your manifest or specific views.
- Optimize Animations: Use property animations and avoid overusing complex animations that can strain the GPU.
10. Stay Updated with Best Practices
The Android ecosystem is constantly evolving, with new tools and best practices emerging regularly:
- Follow Official Guidelines: Regularly consult the Android Developers’ documentation to stay informed about the latest performance optimization techniques.
- Participate in Developer Communities: Engage with forums, attend webinars, and participate in developer communities to exchange knowledge and stay updated on industry trends.
- Continuously Refactor and Improve: Regularly review and refactor your code to incorporate new optimization strategies and remove outdated practices.
Conclusion
Optimizing the performance of your Android apps is a multifaceted endeavor that requires attention to detail across various aspects of development. From efficient layout design and memory management to asynchronous programming and network optimization, each element plays a crucial role in ensuring a fast and responsive user experience. By implementing the tips outlined above and staying committed to best practices, you can create Android applications that not only meet but exceed user expectations, leading to higher engagement, better reviews, and greater success in the competitive app marketplace.
Remember, performance optimization is an ongoing process. Continuously monitor your app’s performance, gather user feedback, and stay informed about the latest advancements in Android development to maintain and enhance your app’s efficiency and responsiveness over time.